Base Program to understand C programming
*Program to show any Written sentence
#include<stdio.h>
#include<conio.h>
void main()
{
printf(" Hello welcome to C Programming");
getch();
}
*Program to enter a number and display numbers:-
#include <stdio.h>
#include <conio.h>
int main()
{
int number;
printf("Enter an integer: ");
// reads and stores input
scanf("%d", &number);
// displays output
printf("You entered: %d", number);
getch();
return 0;
}
1 *C Program to add two numbers:-
#include <stdio.h>
#include <conio.h>
int main()
{
int number1, number2, sum;
printf("Enter two integers: ");
scanf("%d %d", &number1, &number2);
// calculating sum
sum = number1 + number2;
printf("%d + %d = %d", number1, number2, sum);
getch();
return 0;
}
*Program to Multiply Two Numbers
#include <stdio.h>
#include <conio.h>
int main()
{
double a, b, product;
printf("Enter two numbers: ");
scanf("%lf %lf", &a, &b);
// Calculating product
product = a * b;
// Result up to 2 decimal point is displayed using %.2lf
printf("Product = %.2lf", product);
getch();
return 0;
}
*Program to enter a number and display numbers:-
#include <stdio.h>
#include <conio.h>
int main()
{
int number;
printf("Enter an integer: ");
// reads and stores input
scanf("%d", &number);
// displays output
printf("You entered: %d", number);
getch();
return 0;
}
1 *C Program to add two numbers:-
#include <stdio.h>
#include <conio.h>
int main()
{
int number1, number2, sum;
printf("Enter two integers: ");
scanf("%d %d", &number1, &number2);
// calculating sum
sum = number1 + number2;
printf("%d + %d = %d", number1, number2, sum);
getch();
return 0;
}
*Program to Multiply Two Numbers
#include <stdio.h>
#include <conio.h>
int main()
{
double a, b, product;
printf("Enter two numbers: ");
scanf("%lf %lf", &a, &b);
// Calculating product
product = a * b;
// Result up to 2 decimal point is displayed using %.2lf
printf("Product = %.2lf", product);
getch();
return 0;
}
2 * Program to Print ASCII Value
#include <stdio.h>
#include <conio.h>
int main()
{
char c;
printf("Enter a character: ");
scanf("%c", &c);
// %d displays the integer value of a character
// %c displays the actual character
printf("ASCII value of %c = %d", c, c);
getch();
return 0;
}
BY THIS METHOD YOU'LL GET ALL ASCII VALUES LIKE THIS :-
Enter a character: G
ASCII value of G = 71
In this program, the user is asked to enter a character. The character is stored in variable c.
When %d format string is used, 71 (the ASCII value of G) is displayed.
When %c format string is used, 'G' itself is displayed.
*Program to Compute Quotient and Remainder
#include <stdio.h>
#include <conio.h>
int main()
{
int dividend, divisor, quotient, remainder;
printf("Enter dividend: ");
scanf("%d", ÷nd);
printf("Enter divisor: ");
scanf("%d", &divisor);
// Computes quotient
quotient = dividend / divisor;
// Computes remainder
remainder = dividend % divisor;
printf("Quotient = %d\n", quotient);
printf("Remainder = %d", remainder);
getch();
return 0;
}
*Program to Find the Size of Variables
#include<stdio.h>
#include<conio.h>
int main()
{
int intType;
float floatType;
double doubleType;
char charType;
// sizeof evaluates the size of a variable
printf("Size of int: %ld bytes\n", sizeof(intType));
printf("Size of float: %ld bytes\n", sizeof(floatType));
printf("Size of double: %ld bytes\n", sizeof(doubleType));
printf("Size of char: %ld byte\n", sizeof(charType));
getch();
return 0;
}
3 *Swap Numbers Using Temporary Variable
#include<stdio.h>
#include<conio.h>
int main()
{
double first, second, temp;
printf("Enter first number: ");
scanf("%lf", &first);
printf("Enter second number: ");
scanf("%lf", &second);
// Value of first is assigned to temp
temp = first;
// Value of second is assigned to first
first = second;
// Value of temp (initial value of first) is assigned to second
second = temp;
printf("\nAfter swapping, firstNumber = %.2lf\n", first);
printf("After swapping, secondNumber = %.2lf", second);
return 0;
}
4 *Program to Check Even or Odd
#include <stdio.h>
#include <conio.h>
int main()
{
int num;
printf("Enter an integer: ");
scanf("%d", &num);
// True if num is perfectly divisible by 2
if(num % 2 == 0)
printf("%d is even.", num);
else
printf("%d is odd.", num);
getch();
return 0;
}
5 *Program to Check Odd or Even Using the Ternary Operator
#include <stdio.h>
#include <conio.h>
int main()
{
int num;
printf("Enter an integer: ");
scanf("%d", &num);
(num % 2 == 0) ? printf("%d is even.", num) : printf("%d is odd.", num);
getch();
return 0;
}
6 * C Program to Find the Largest Number Among Three Numbers
#include <stdio.h>
#include <conio.h>
int main()
{
char c;
printf("Enter a character: ");
scanf("%c", &c);
// %d displays the integer value of a character
// %c displays the actual character
printf("ASCII value of %c = %d", c, c);
getch();
return 0;
}
BY THIS METHOD YOU'LL GET ALL ASCII VALUES LIKE THIS :-
Enter a character: G
ASCII value of G = 71
In this program, the user is asked to enter a character. The character is stored in variable c.
When %d format string is used, 71 (the ASCII value of G) is displayed.
When %c format string is used, 'G' itself is displayed.
*Program to Compute Quotient and Remainder
#include <stdio.h>
#include <conio.h>
int main()
{
int dividend, divisor, quotient, remainder;
printf("Enter dividend: ");
scanf("%d", ÷nd);
printf("Enter divisor: ");
scanf("%d", &divisor);
// Computes quotient
quotient = dividend / divisor;
// Computes remainder
remainder = dividend % divisor;
printf("Quotient = %d\n", quotient);
printf("Remainder = %d", remainder);
getch();
return 0;
}
*Program to Find the Size of Variables
#include<stdio.h>
#include<conio.h>
int main()
{
int intType;
float floatType;
double doubleType;
char charType;
// sizeof evaluates the size of a variable
printf("Size of int: %ld bytes\n", sizeof(intType));
printf("Size of float: %ld bytes\n", sizeof(floatType));
printf("Size of double: %ld bytes\n", sizeof(doubleType));
printf("Size of char: %ld byte\n", sizeof(charType));
getch();
return 0;
}
3 *Swap Numbers Using Temporary Variable
#include<stdio.h>
#include<conio.h>
int main()
{
double first, second, temp;
printf("Enter first number: ");
scanf("%lf", &first);
printf("Enter second number: ");
scanf("%lf", &second);
// Value of first is assigned to temp
temp = first;
// Value of second is assigned to first
first = second;
// Value of temp (initial value of first) is assigned to second
second = temp;
printf("\nAfter swapping, firstNumber = %.2lf\n", first);
printf("After swapping, secondNumber = %.2lf", second);
return 0;
}
4 *Program to Check Even or Odd
#include <stdio.h>
#include <conio.h>
int main()
{
int num;
printf("Enter an integer: ");
scanf("%d", &num);
// True if num is perfectly divisible by 2
if(num % 2 == 0)
printf("%d is even.", num);
else
printf("%d is odd.", num);
getch();
return 0;
}
5 *Program to Check Odd or Even Using the Ternary Operator
#include <stdio.h>
#include <conio.h>
int main()
{
int num;
printf("Enter an integer: ");
scanf("%d", &num);
(num % 2 == 0) ? printf("%d is even.", num) : printf("%d is odd.", num);
getch();
return 0;
}
6 * C Program to Find the Largest Number Among Three Numbers
#include<stdio.h>
#include<conio.h>
int main()
{
int a,b,c;
clrscr();
printf("\n Enter three numbers : ");
scanf("%d%d%d",&a,&b,&c);
(((a>b)?printf("\n A is bigger "):printf("\n B is bigger
")&&((a>c)?printf("\n A is bigger"):printf("\n C is bigger"));
getch();
return 0;
}
C Program to Find the Largest Number Among Three Numbers
7. Example 3: Using Nested if...else
#include <stdio.h>
#include <conio.h>
int main()
{
double n1, n2, n3;
printf("Enter three numbers: ");
scanf("%lf %lf %lf", &n1, &n2, &n3);
if (n1 >= n2) {
if (n1 >= n3)
printf("%.2lf is the largest number.", n1);
else
printf("%.2lf is the largest number.", n3);
} else {
if (n2 >= n3)
printf("%.2lf is the largest number.", n2);
else
printf("%.2lf is the largest number.", n3);
}
getch();
return 0;
}
8. Write a program to reverse a number:
#include <stdio.h>
#include<conio.h>
int reversDigits(int num)
{
int rev_num = 0;
while(num > 0)
{
rev_num = rev_num*10 + num%10;
num = num/10;
}
return rev_num;
}
/*Driver program to test reversDigits*/
int main()
{
int num = 4562;
printf("Reverse of no. is %d", reversDigits(num));
getch();
return 0;
}
9. *C program to find sum of first and last digit of a
number using loop
#include <stdio.h>
#include<conio.h>
int main()
{
int num, sum=0, firstDigit, lastDigit;
clrscr();
/* Input a number from user */
printf("Enter any number to find sum of first and last
digit: ");
scanf("%d", &num);
/* Find last digit to sum */
lastDigit = num % 10;
/* Copy num to first digit */
firstDigit = num;
/* Find the first digit by dividing num by 10 until
first digit is left */
while(num >= 10)
{
num = num / 10;
}
firstDigit = num;
/* Find sum of first and last digit*/
sum = firstDigit + lastDigit;
printf("Sum of first and last digit = %d", sum);
getch();
return 0;
}
10. * Program to calculate sum of all 5 digits entered
as input from keyboard
#include <stdio.h>
#include<conio.h>
int main()
{
int n, t, sum = 0, remainder;
clrscr();
printf("Enter an integer\n");
scanf("%d", &n);
t = n;
while (t != 0)
{
remainder = t % 10;
sum= sum +
remainder;
t= t / 10;
}
printf("Sum of digits of %d = %d\n", n, sum);
getch();
return 0;
}
*Program to Check Vowel or consonant
#include <stdio.h>
#include <conio.h>
int main()
{
char c;
int lowercase_vowel, uppercase_vowel;
printf("Enter an alphabet: ");
scanf("%c", &c);
// evaluates to 1 if variable c is a lowercase vowel
lowercase_vowel = (c == 'a' || c == 'e' || c == 'i' || c == 'o' || c == 'u');
// evaluates to 1 if variable c is a uppercase vowel
uppercase_vowel = (c == 'A' || c == 'E' || c == 'I' || c == 'O' || c == 'U');
// evaluates to 1 (true) if c is a vowel
if (lowercase_vowel || uppercase_vowel)
printf("%c is a vowel.", c);
else
printf("%c is a consonant.", c);
getch();
return 0;
}
#include <stdio.h>
#include <conio.h>
int main()
{
char c;
int lowercase_vowel, uppercase_vowel;
printf("Enter an alphabet: ");
scanf("%c", &c);
// evaluates to 1 if variable c is a lowercase vowel
lowercase_vowel = (c == 'a' || c == 'e' || c == 'i' || c == 'o' || c == 'u');
// evaluates to 1 if variable c is a uppercase vowel
uppercase_vowel = (c == 'A' || c == 'E' || c == 'I' || c == 'O' || c == 'U');
// evaluates to 1 (true) if c is a vowel
if (lowercase_vowel || uppercase_vowel)
printf("%c is a vowel.", c);
else
printf("%c is a consonant.", c);
getch();
return 0;
}
To understand this example, you should have the knowledge of the
following C programming topics:
C if...else Statement
In this tutorial, you will learn about if statement (including if...else and nested if..else) in C programming with the help of examples.
C if Statement
The syntax of the if statement in C programming is:
if (test expression)
{
// statements to be executed if the test expression is true
}
How if statement works?
The if statement evaluates the test expression inside the parenthesis ().
If the test expression is evaluated to true, statements inside the body of if are executed.
If the test expression is evaluated to false, statements inside the body of if are not executed.
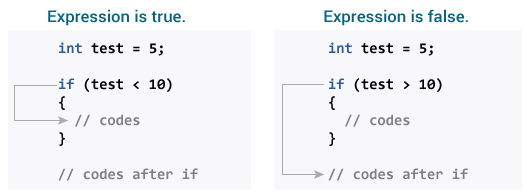
Example 1: if statement
// Program to display a number if it is negative
#include <stdio.h>
int main()
{
int number;
printf("Enter an integer: ");
scanf("%d", &number);
// true if number is less than 0
if (number < 0) {
printf("You entered %d.\n", number);
}
printf("The if statement is easy.");
return 0;
}
Output 1
Enter an integer: -2
You entered -2.
The if statement is easy.
When the user enters -2, the test expression number<0 is evaluated to true. Hence, You entered -2 is displayed on the screen.
Output 2
Enter an integer: 5
The if statement is easy.
When the user enters 5, the test expression number<0 is evaluated to false and the statement inside the body of if is not executed
C if...else Statement
The if statement may have an optional else block. The syntax of the if..else statement is:
if (test expression)
{
// statements to be executed if the test expression is true
}
else
{
// statements to be executed if the test expression is false
}
Example : Using if Statement
#include <stdio.h>
#include <conio.h>
int main()
{
double n1, n2, n3;
printf("Enter three different numbers: ");
scanf("%lf %lf %lf", &n1, &n2, &n3);
// if n1 is greater than both n2 and n3, n1 is the largest
if (n1 >= n2 && n1 >= n3)
printf("%.2f is the largest number.", n1);
// if n2 is greater than both n1 and n3, n2 is the largest
if (n2 >= n1 && n2 >= n3)
printf("%.2f is the largest number.", n2);
// if n3 is greater than both n1 and n2, n3 is the largest
if (n3 >= n1 && n3 >= n2)
printf("%.2f is the largest number.", n3);
getch();
return 0;
}
C Program to Find the Largest Number Among Three Numbers
Example 2: Using if...else Ladder
#include <stdio.h>
#include <conio.h>
int main()
{
double n1, n2, n3;
printf("Enter three numbers: ");
scanf("%lf %lf %lf", &n1, &n2, &n3);
// if n1 is greater than both n2 and n3, n1 is the largest
if (n1 >= n2 && n1 >= n3)
printf("%.2lf is the largest number.", n1);
// if n2 is greater than both n1 and n3, n2 is the largest
else if (n2 >= n1 && n2 >= n3)
printf("%.2lf is the largest number.", n2);
// if both above conditions are false, n3 is the largest
else
printf("%.2lf is the largest number.", n3);
getch();
return 0;
}
- C Programming Operators
- C if...else Statement
C if...else Statement
In this tutorial, you will learn about if statement (including if...else and nested if..else) in C programming with the help of examples.
C if Statement
The syntax of the if statement in C programming is:
if (test expression)
{
// statements to be executed if the test expression is true
}
How if statement works?
The if statement evaluates the test expression inside the parenthesis ().
If the test expression is evaluated to true, statements inside the body of if are executed.
If the test expression is evaluated to false, statements inside the body of if are not executed.
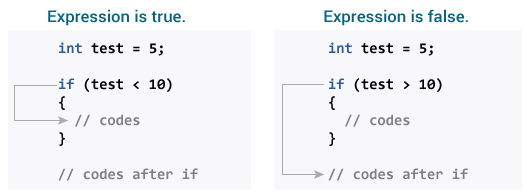
Example 1: if statement
// Program to display a number if it is negative
#include <stdio.h>
int main()
{
int number;
printf("Enter an integer: ");
scanf("%d", &number);
// true if number is less than 0
if (number < 0) {
printf("You entered %d.\n", number);
}
printf("The if statement is easy.");
return 0;
}
Output 1
Enter an integer: -2
You entered -2.
The if statement is easy.
When the user enters -2, the test expression number<0 is evaluated to true. Hence, You entered -2 is displayed on the screen.
Output 2
Enter an integer: 5
The if statement is easy.
When the user enters 5, the test expression number<0 is evaluated to false and the statement inside the body of if is not executed
C if...else Statement
The if statement may have an optional else block. The syntax of the if..else statement is:
if (test expression)
{
// statements to be executed if the test expression is true
}
else
{
// statements to be executed if the test expression is false
}
Example : Using if Statement
#include <stdio.h>
#include <conio.h>
int main()
{
double n1, n2, n3;
printf("Enter three different numbers: ");
scanf("%lf %lf %lf", &n1, &n2, &n3);
// if n1 is greater than both n2 and n3, n1 is the largest
if (n1 >= n2 && n1 >= n3)
printf("%.2f is the largest number.", n1);
// if n2 is greater than both n1 and n3, n2 is the largest
if (n2 >= n1 && n2 >= n3)
printf("%.2f is the largest number.", n2);
// if n3 is greater than both n1 and n2, n3 is the largest
if (n3 >= n1 && n3 >= n2)
printf("%.2f is the largest number.", n3);
getch();
return 0;
}
C Program to Find the Largest Number Among Three Numbers
Example 2: Using if...else Ladder
#include <stdio.h>
#include <conio.h>
int main()
{
double n1, n2, n3;
printf("Enter three numbers: ");
scanf("%lf %lf %lf", &n1, &n2, &n3);
// if n1 is greater than both n2 and n3, n1 is the largest
if (n1 >= n2 && n1 >= n3)
printf("%.2lf is the largest number.", n1);
// if n2 is greater than both n1 and n3, n2 is the largest
else if (n2 >= n1 && n2 >= n3)
printf("%.2lf is the largest number.", n2);
// if both above conditions are false, n3 is the largest
else
printf("%.2lf is the largest number.", n3);
getch();
return 0;
}
Awesome work
ReplyDeleteThank u , stay tuned
ReplyDelete